Represents a Message Button.
These buttons are located below the message in
ActionRows
.
Each button has either a custom_id
or URL attached.
The id has to be provided by the user and can be used to identify the button in the ButtonInteractionEvent
.
Example Usage
public class HelloBot extends ListenerAdapter {
public void onSlashCommandInteraction(SlashCommandInteractionEvent event) {
if (event.getName().equals("hello")) {
event.reply("Click the button to say hello")
.addActionRow(
Button.primary("hello", "Click Me"), // Button with only a label
Button.success("emoji", Emoji.fromMarkdown("<:minn:245267426227388416>"))) // Button with only an emoji
.queue();
} else if (event.getName().equals("info")) {
event.reply("Click the buttons for more info")
.addActionRow( // link buttons don't send events, they just open a link in the browser when clicked
Button.link("https://github.com/discord-jda/JDA", "GitHub")
.withEmoji(Emoji.fromMarkdown("<:github:849286315580719104>")), // Link Button with label and emoji
Button.link("https://docs.jda.wiki/", "Javadocs")) // Link Button with only a label
.queue();
}
}
public void onButtonInteraction(ButtonInteractionEvent event) {
if (event.getComponentId().equals("hello")) {
event.reply("Hello :)").queue();
}
}
}
To see what each button looks like here is an example cheatsheet:
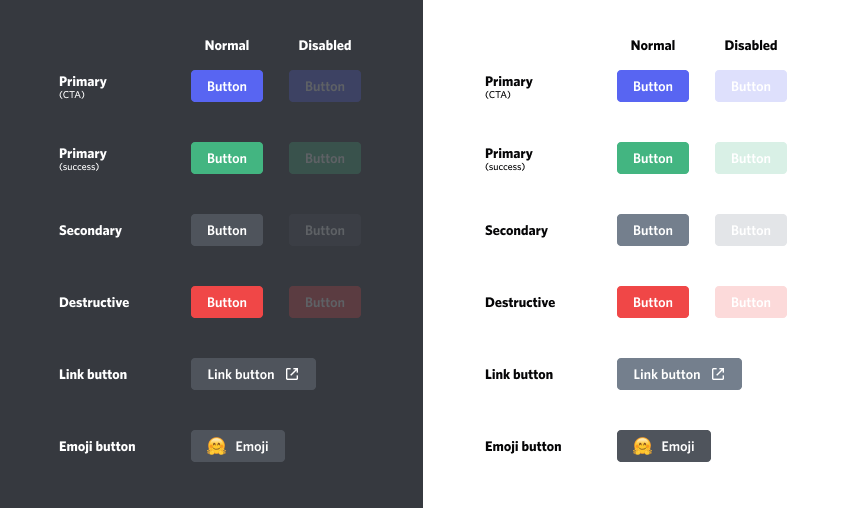